Introduction to the map() Function: Behind the Scenes
๐ Welcome
In this tutorial you will learn how to work with the built-in
map()
function. Let’s begin.๐น Purpose and Syntax
The
map()
function applies a function that is passed as argument to every element of an iterable.
๐ก Tip: An iterable is an object capable of returning its members one at a time (for example: lists, tuples, dictionaries, and strings). They can be used in for loops.
The
map()
function takes two parameters (in this order):- The function that we want to apply to each item of the iterable.
- The iterable.
๐ธ Example
If we have this line of code:
map(f, [1, 2, 3, 4, 5])
Where
f
is a function that is already defined in the program, this is what happens behind the scenes:
The function
f
is called with the first element of the iterable as the argument (in this case, 1). That function call is completed and a value is returned (if the function returns a value).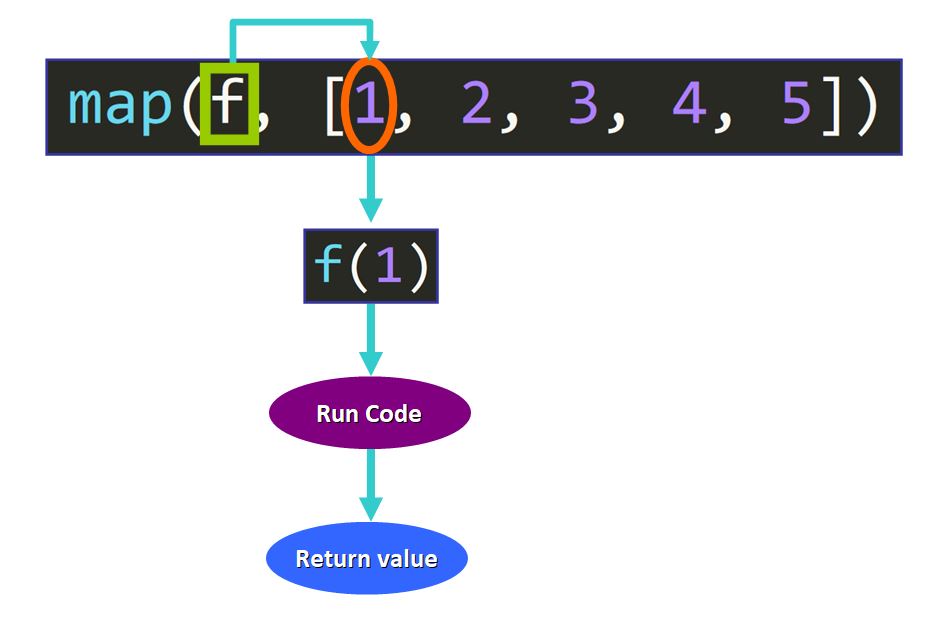
The same process is repeated with all the items of the iterable. In this case, with the integers 2, 3, 4, and 5.
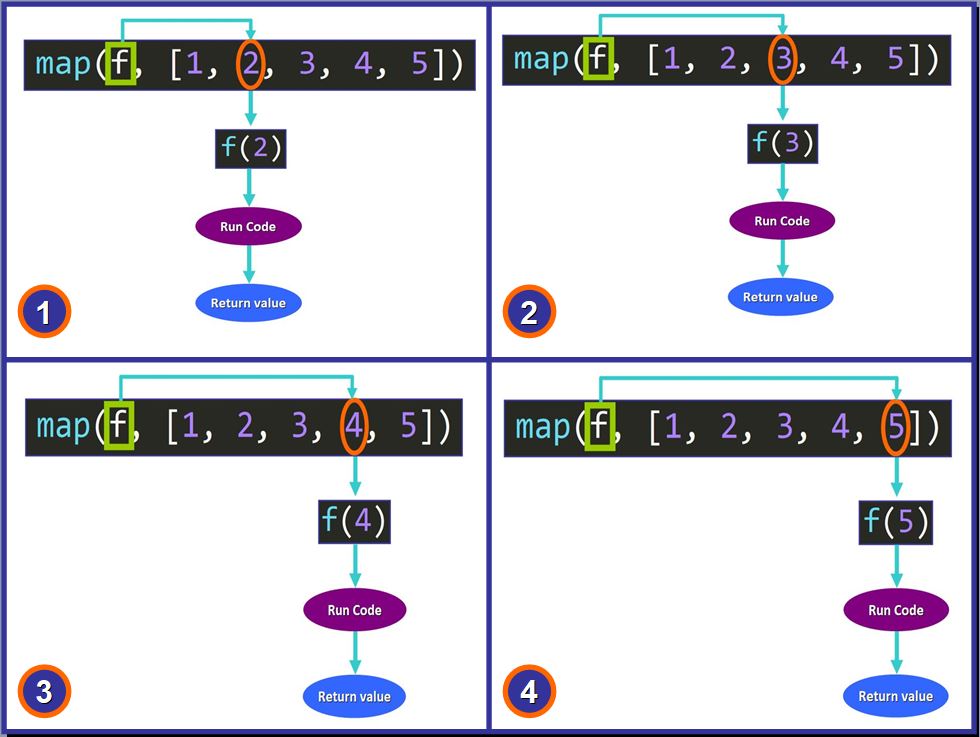
The values returned are kept in a map object, which is an iterator (a type of object that is used to access the elements of an iterable one at a time. It is commonly used in loops). (More info on map() in the Python Documentation).
Here we have an example:
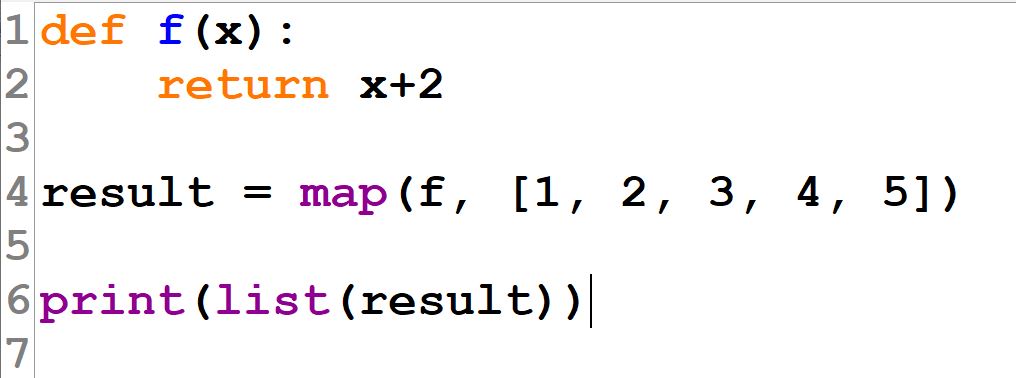
๐ก Tip: In this tutorial, we convert (cast) the result into a list to see its content. You also cast it to a tuple.
The output is:
[3, 4, 5, 6, 7]
This is what happened “behind the scenes”:
- f(1) returned 1 + 2 = 3 --> first element of the iterator.
- f(2) returned 2 + 2 = 4 --> second element of the iterator.
- f(3) returned 3 + 2 = 5 --> third element of the iterator.
- f(4) returned 4 + 2 = 6 --> fourth element of the iterator.
- f(5) returned 5 + 2 = 7 --> fifth element of the iterator.
๐น map() with Two Iterables
That was interesting, right? But you might be asking…
What if the function takes more than one argument?
In that case, we can pass more than one iterable and the items at corresponding positions of the iterables will be passed as arguments simultaneously.
Let’s see an example where the function takes two arguments:
map(f, [1, 2, 3], [4, 5, 6])
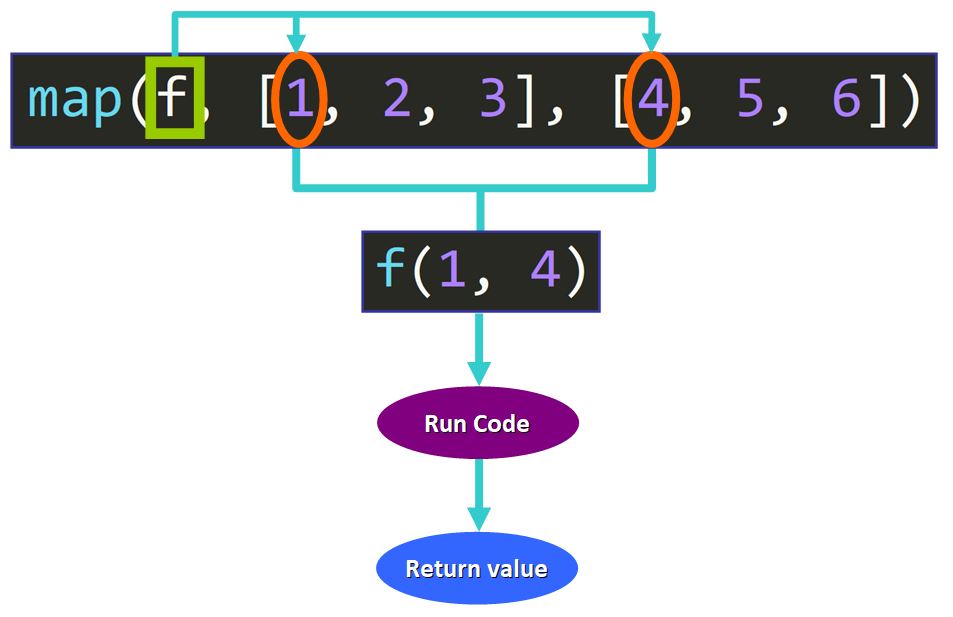
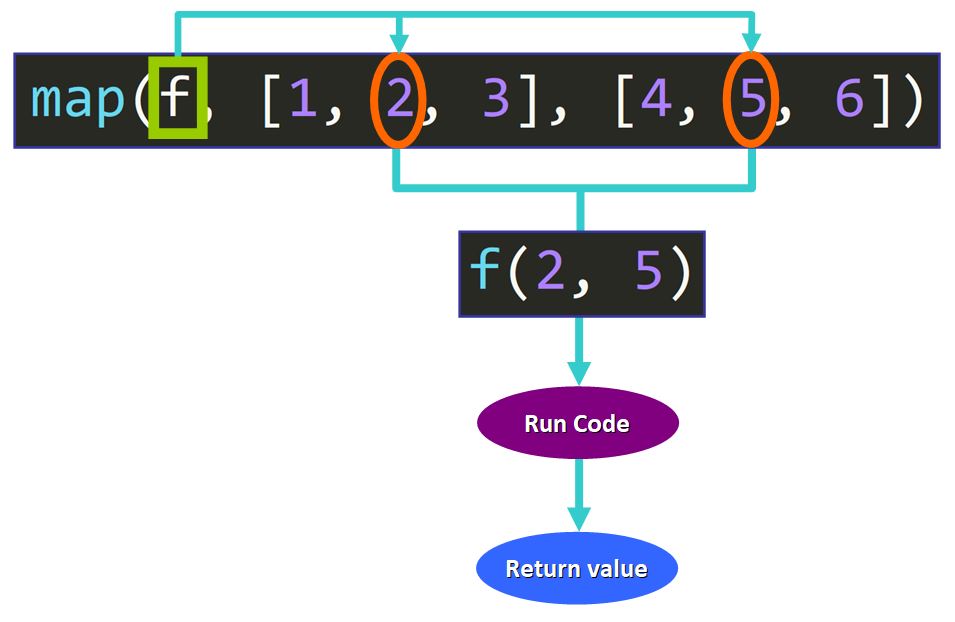
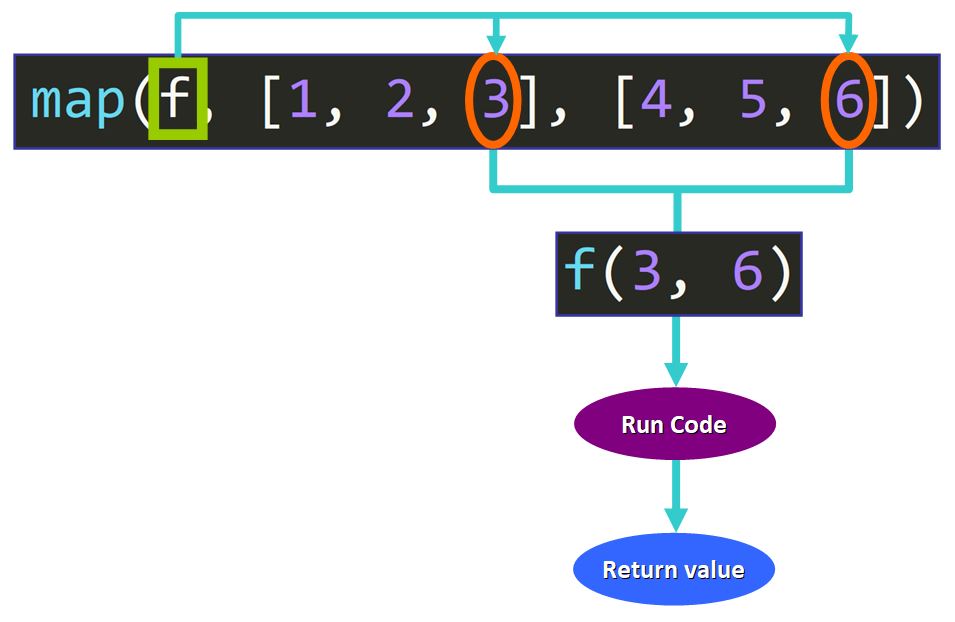
Here we have the code:
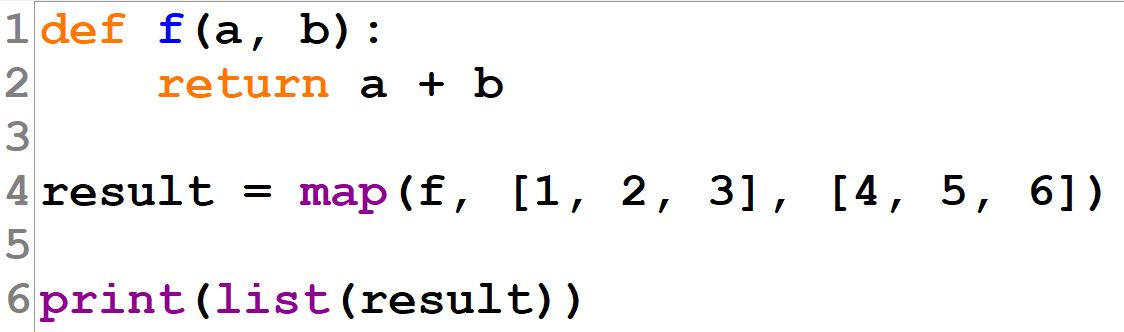
The output is:
[5, 7, 9]
The result is an iterator with the values returned by the function calls (in the order of execution of the function calls).
This is what happened “behind the scenes”:
- f(1, 4) returned 1 + 4 = 5 --> first element of the iterator.
- f(2, 5) returned 2 + 5 = 7 --> second element of the iterator.
- f(3, 6) returned 3 + 6 = 9 --> third element of the iterator.
Let’s see another example.
๐ธ Example with Strings
So far, we have worked with integers. Now let’s work with strings and a function that returns boolean values (
True
/False
).
Here we have the iterables (lists of strings) and the definition of the function that we will use in the example. The function returns
True
if the two strings have the same length (number of characters) and False
if they don't.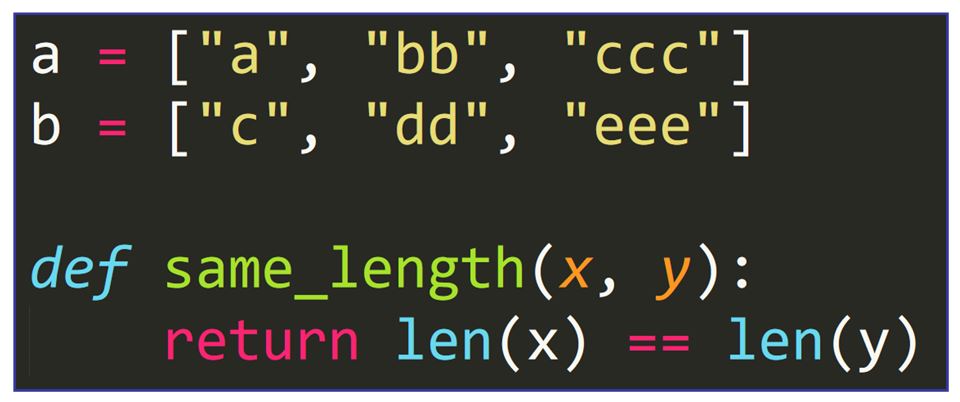
When we call the
map()
function passing the function and the two iterables as arguments, the strings at the corresponding positions of the lists are passed simultaneously as arguments to the function same_length
:
The first function call returns
True
because the two strings have the same (1).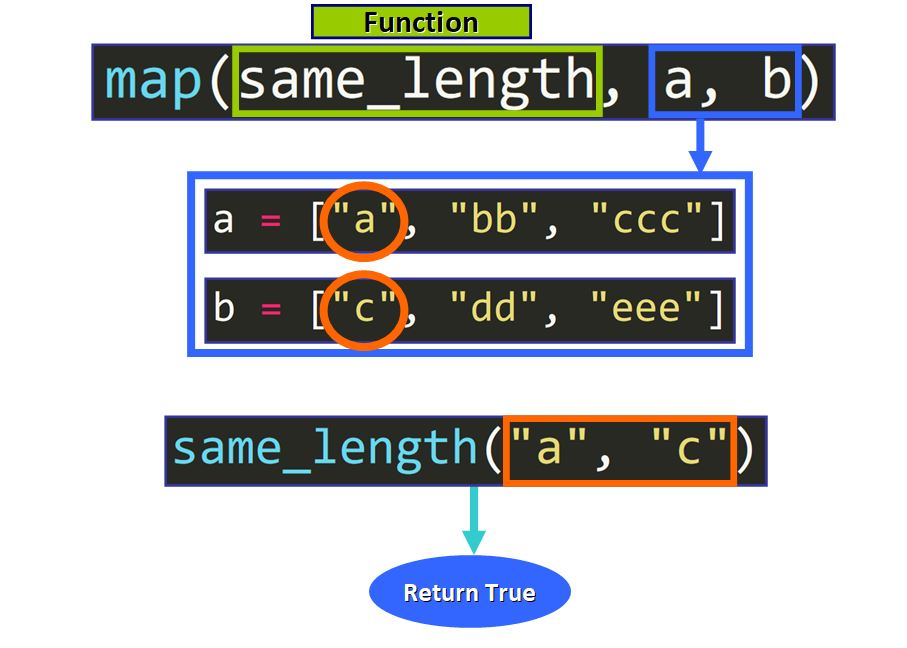
The second function call returns
True
as well because the two strings have the same length (2):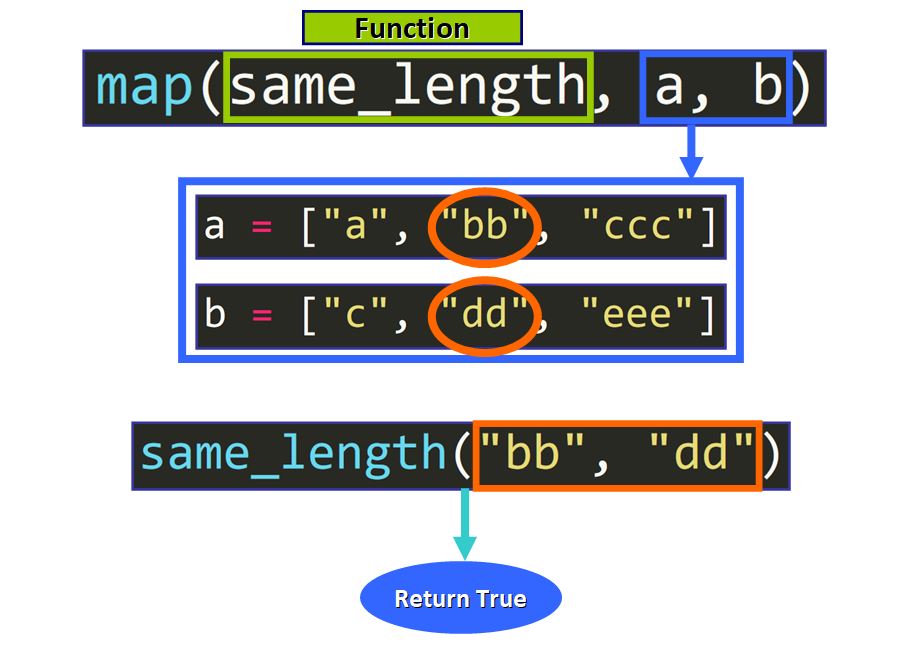
Finally, the third function call returns
True
because they have the two strings same length (3).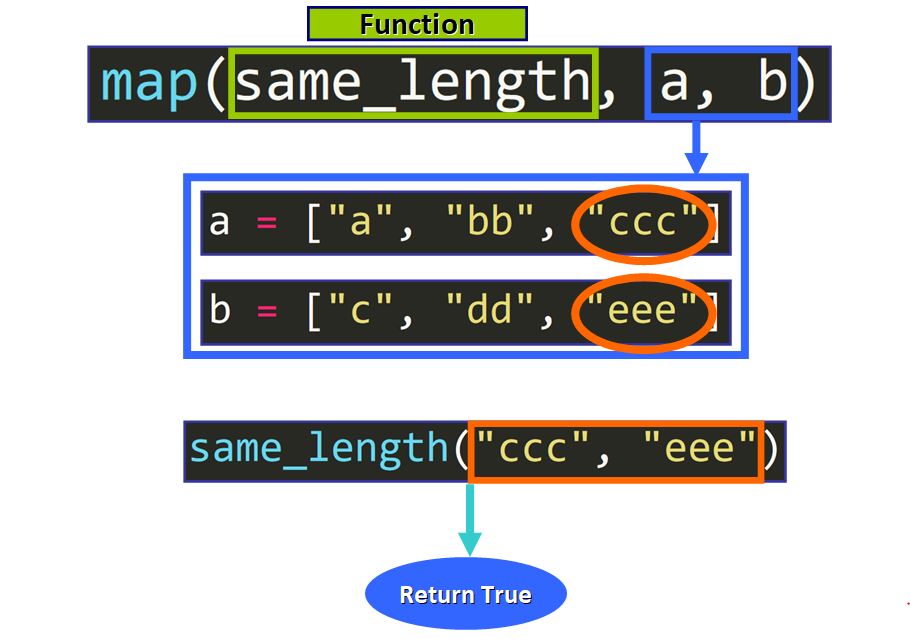
This is the code:
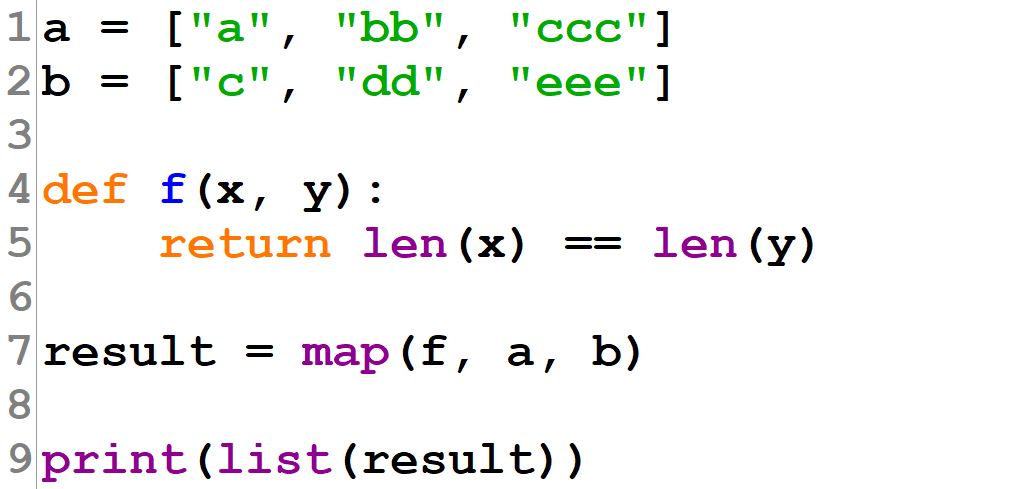
This is the output:
[True, True, True]
The truth values were returned and added to the iterator.
You can see that
map()
is a very powerful built-in function that lets you apply the power of a function to several elements in one line.
๐ You can use the iterator returned by map() in a for loop just like you use range(). There will be one iteration per element.
>>> def f(x):
return x*2
>>> for i in map(f, [2, 3, 4]):
print(i)
4
6
8
I hope you liked this tutorial and found it helpful. ๐
Please do not hesitate to ask if you have any questions. Community TAs and your classmates will be very glad to help you on the discussion forums.
Estefania.
Hi, In what order am i supposed to follow this tutorial?
ReplyDelete