Try-Except-Else-Finally: Behind the Scenes
π Try-Except-Else-Finally: Behind the Scenes
Hi! Welcome to this short tutorial where you will learn how exception handling works behind the scenes when you use try-except-else-finally blocks.
Let’s begin! π
This is the basic structure used to handle exceptions in Python (diagram below).
We have four blocks:
- try: this is the code that you will try to run. What happens after this depends on whether an exception was thrown or not.
- except: this block handles the exception if it occurs when the try block is executed.
- else: this code block runs only if no exceptions were thrown in the try block.
- finally: this block always runs, even if there were exceptions in the try block. As noted in the lecture, this block if very helpful for “clean up code” such as closing files.
π‘ Note: the else and finally blocks are optional. If there is a finally block, else must be included before the finally block.
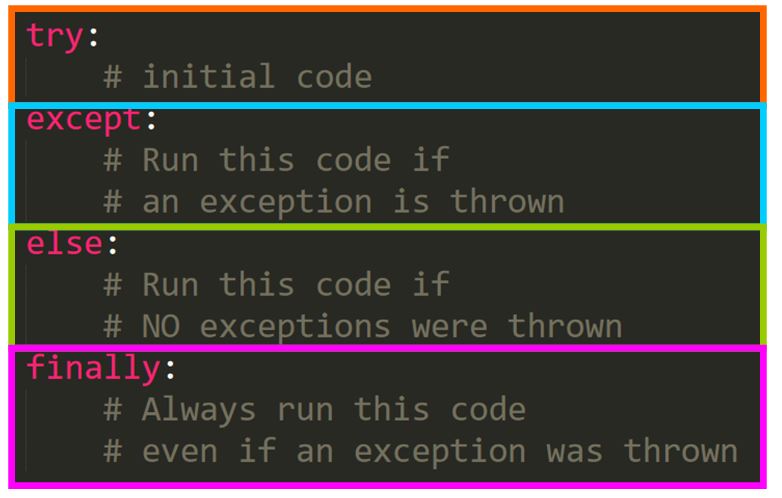
π© Exception Thrown in the Try Block
Let’s see what happens if an exception is thrown in the try block.
First, the code in the try block is executed. An exception is thrown. The program would immediately “jump” to the except clause, interrupting the execution of the try block. The except block would run and then the finally clause would be executed.
The sequence would be:
try -> except -> finally
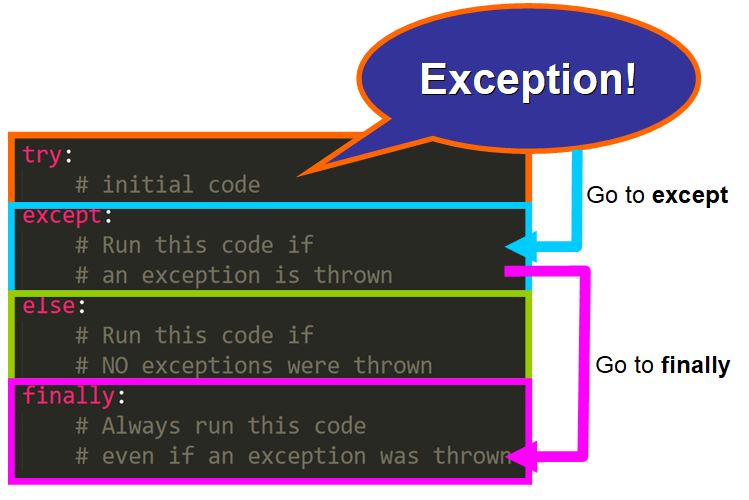
Here you can see an example of this in the Python shell:
Trying to divide by 0 throws an exception in the try block. The program “jumps” to the except block and executes it. Once it’s completed, the program “jumps” again to the finally block.
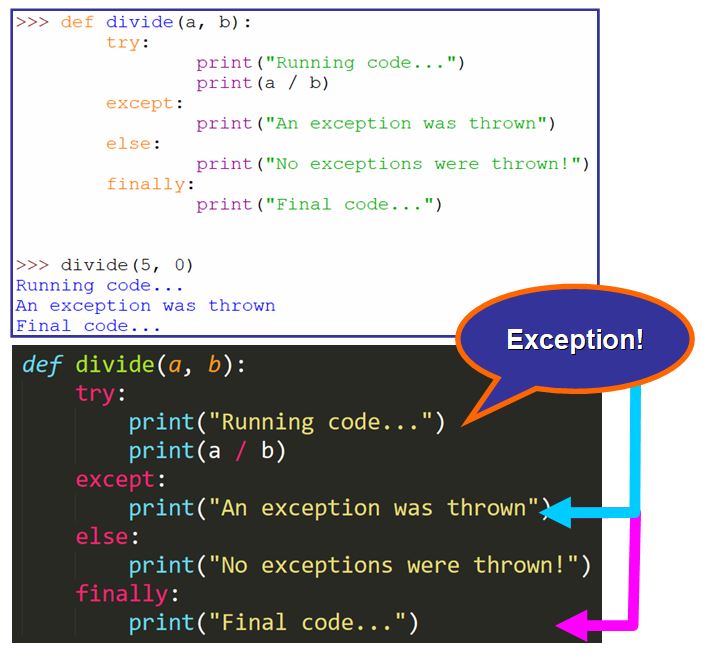
π© No Exception Thrown in the Try Block
If the code in the try block runs without throwing any exceptions, the program “jumps” to the else block, runs that code block and “jumps” again to the finally block.
The sequence would be:
try -> else -> finally
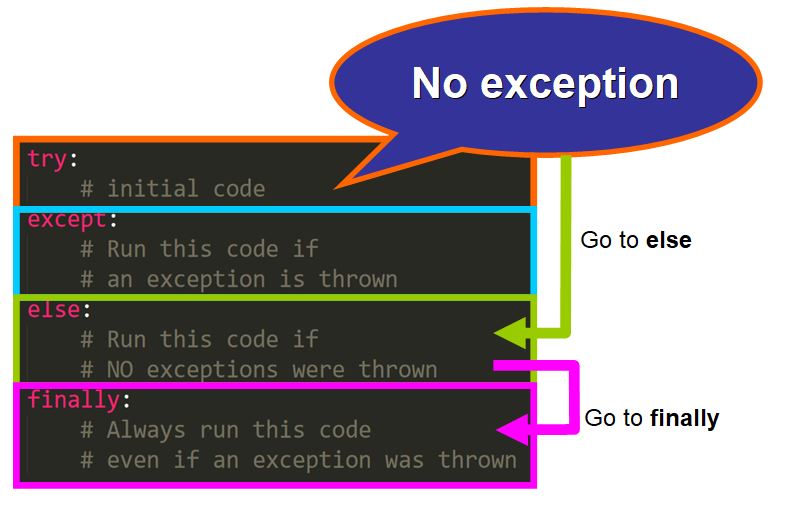
Here you can see an example of this. The try block is executed first, then the flow of execution “jumps” to the else block, and the finally clause is executed last.
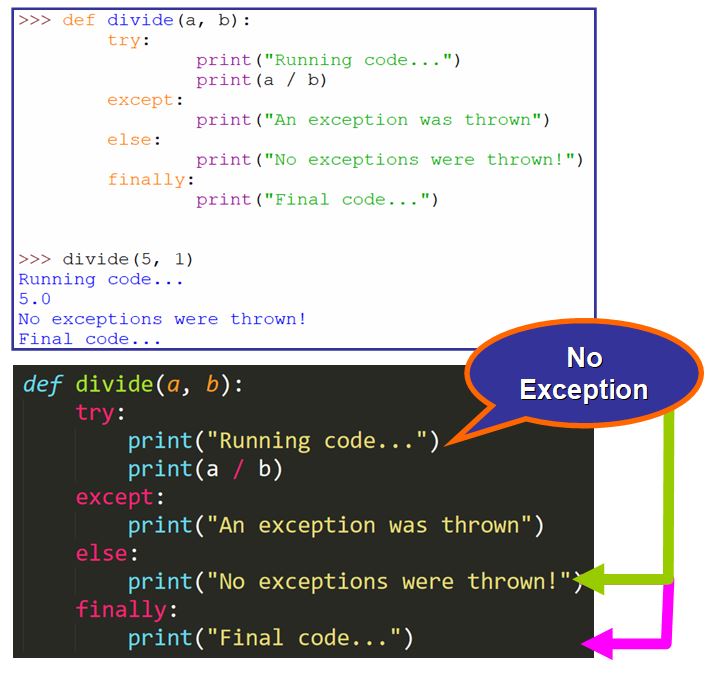
π© Using "Exception as e" in the Except Block
In the except block, you can access the information of the exception that was thrown in the try block by using this syntax:
except <exception_type> as <variable_to_hold_exception>:
In the example below, the function specifically handles the IndexError exception in the except block and assigns it to the variable
e
that you can use within the except block. In this case, I decided to print the message of the exception, which you can see in the second image below.
π‘ Note: you can catch a specific type of exception such as IndexError or you can use a more generic form, Exception, which will catch most types of exceptions. This is because most of the built-in exceptions that you will use are subtypes of
Exception
(you can think of Exception as being a general form used to refer to an exception). You can read more about this “hierarchy” in the Python documentation: https://docs.python.org/3/library/exceptions.html#exception-hierarchy .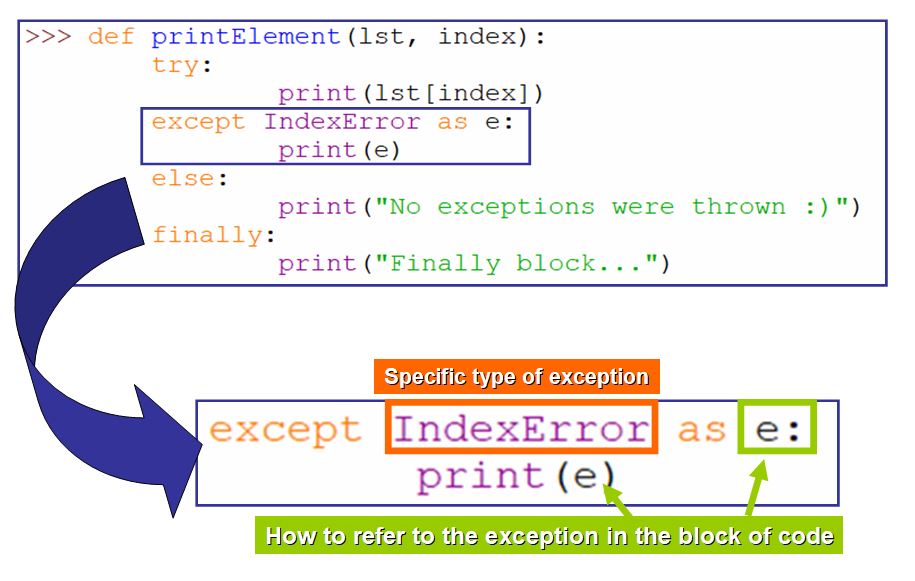
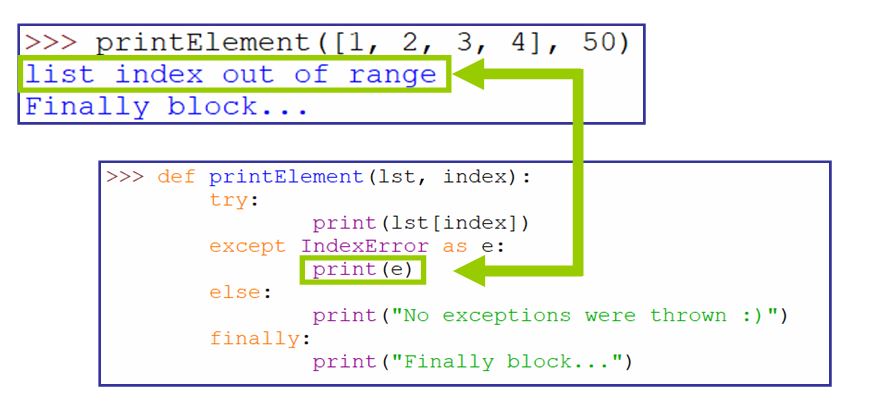
I really hope you liked this tutorial and found it helpful. π I would like to offer you my sincere congratulations for these past weeks of hard work and dedication. π
Please do not hesitate to ask on the discussion forums or right below this post if you have any questions. Community TAs and your classmates will be there to help you.
Estefania.
Hello Estefania, thank you for the clear explanation!
ReplyDeleteDo you know a set of exercises that are useful for learning Try - Except, and also assert?
Also, do you find it useful for things other than preventing/fiding bugs? Like instead of doing conditionals if-else statements, sometines I can do Try-Except?
Thank you
This comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThnx for the nice explanation.
ReplyDeleteDo I understand this correctly, that an else block optional is; but if finally is used, one must be included? With no exception, the code after try will run anyway, so why use else at all?! Just to have a finally?
Thnx for the nice explanation.
ReplyDeleteDo I understand this correctly, that an else block optional is; but if finally is used, one must be included? With no exception, the code after try will run anyway, so why use else at all?! Just to have a finally!