Lists vs. Tuples: Behind the Scenes
Hi, welcome to this new tutorial! π We will dive into the similarities and differences between lists and tuples. It’s very important that you understand their key differences, so let’s begin! π
π Syntax and Structure
- The syntax used to define lists and tuples is very similar, but they differ in a very important aspect: lists are enclosed in square brackets [ ] and tuples are enclosed in parentheses ( ).
- They both contain values separated by commas and those values can be of different types, so you can include integers, strings, floats, other lists, and even tuples as values. (Lists within lists are called “Nested Lists” and tuples within tuple are called “Nested tuples”).
- They have a structure that is very similar to the grid that we used to work with strings. Each element can be accessed by using its corresponding index, like we did with strings in Weeks 1 and 2. The first value can be accessed using index 0, the second value using index 1, and so on… exactly like strings!
Please take a moment to take a look at these diagrams below to visualize the structure of lists and tuples. π The first diagram shows a list and the second diagrams shows a tuple.
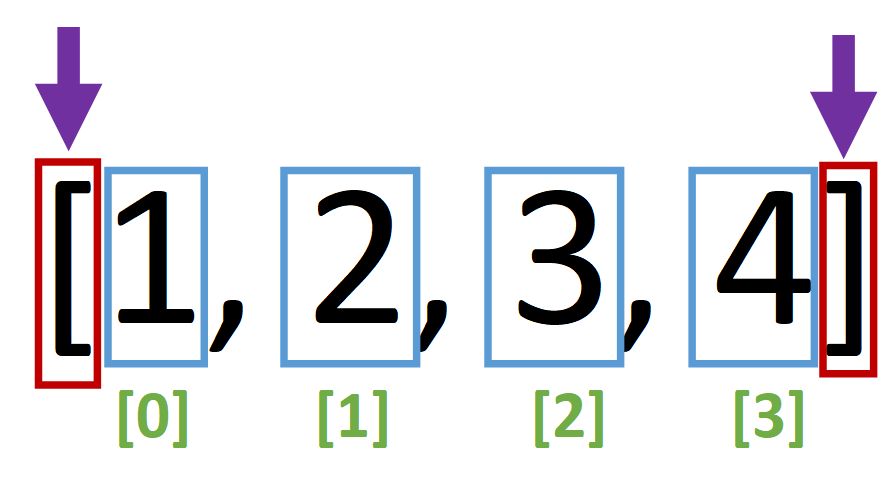
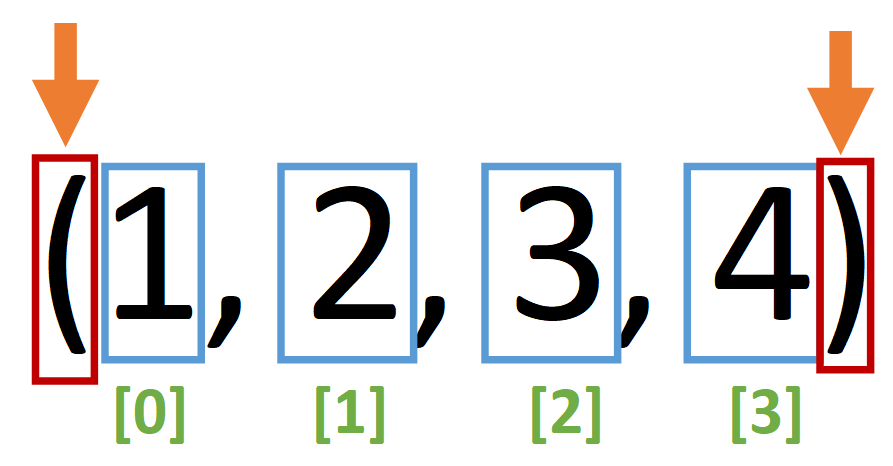
π» Let's Code!
Now let’s see some code: You create a list with [ ] separating the values with a comma and a space after the comma for style purposes to help other developers read your code more easily. We can check the type of this value using type().
>>> list1 = [1, 2, 3, 4]
>>> type(list1)
<class 'list'>
We can do the same with tuples:
>>> tuple1 = (1, 2, 3, 4)
>>> type(tuple1)
>>> <class 'tuple'>
To access a value contained within a list or tuple, we use an index, exactly like we did with strings:
>>> list1[2]
3
>>> tuple1[0]
1
We can also extract a portion of the list or tuple using the same logic and notation that we used with string slicing:
<list_or_tuple>[start:end:step]
and we will obtain a list or tuple as a result (the resulting type, list or tuple, is determined by the original value, if it's a list, the slice will be a list and if it's a tuple, the slice will be a tuple).>>> list1[1:3]
[2, 3]
>>> tuple1[::-1]
(4, 3, 2, 1)
>>> list1[::2]
[1, 3]
π Now… their difference!
So far… everything seems very similar between lists and tuple, right? They only differ in [ ] and ( ).
But I’m sure you must be asking yourself: why would they be different data types if they are so similar? There must be a difference!
You’re right, there is!
Their main difference lies in their mutability, the ability to change their individual values once the tuple or list has been defined.
For example, if you have this list:
>>> list2 = ["a", "b", "c"]
Do you think we could change the character at index 1 for a different string? Let’s say we want to change “b” to “e”. Could we do it?
Yes, we can! We can do it because lists are a Mutable data type. You can simply change this value in the original list without making a copy of the entire list to make this modification.
In contrast, if we have this tuple:
>>> tuple2 = ("a", "b", "c")
We would NOT be able to change this individual value and we would get an error if we tried because tuple is an Immutable data types. You cannot change the original tuple. You could only make a copy of the original tuple with this value modified and that would require tuple concatenation (you will see an example of this below).
π» Let's Code!
Let’s try this in IDLE:
>>> list2 = ["a", "b", "c"]
>>> list2[1] = "e" # Specify the index and the new value
>>> list2
['a', 'e', 'c'] # The value was updated!
Now, if we try to do this with a tuple, we get an error:
>>> tuple2 = ("a", "b", "c")
>>> tuple2[1] = "e" # This throws an error
Traceback (most recent call last):
File "<pyshell#23>", line 1, in <module>
tuple2[1] = "e"
TypeError: 'tuple' object does not support item assignment
>>> tuple2
('a', 'b', 'c') # The value was not changed
π Alternative Approach
To replace this value at index 1, we would need to slice the tuple and concatenate the slice with the new value contained within a new tuple, like this:
>>> tuple2 = tuple2[:1] + ("e",) + tuple2[2:]
This concatenates three tuples: a tuple that goes from index 0 to index 1 (not inclusive), a new tuple with a single element (the new element that we want to include) and a third tuple that goes from index 2 to the end of the tuple. The resulting value is a tuple.
>>> tuple2
('a', 'e', 'c')
Note: In this case, we could have concatenated three tuples with the three elements because the tuple was very simple, but in a more realistic scenario we could use this slicing technique.
We see that it’s changed. Notice that this is NOT the original tuple, it is a new tuple that we created and then assigned to this variable, replacing the old value. Imagine how much time and computations it would take to do this on a very large tuple. This is why we use tuples when we know that the data will not change during the program and we use lists when it’s possible that the values might change.
π‘ Practice
Now it's time for some practice! Try running these examples in IDLE or in your Python IDE to experiment with them a little bit more π
c = [(1, 2, 3), "Week 3", 6.453, [[1, 2, 3, 4], 5], 678, [True, False]]
Exercises:
>>> c[0]
>>> c[3:]
>>> c[3][0][2] (Try this and analyze why you get this a result, it’s really cool! π)
>>> c[3][1]
>>> c[::-2]
>>> c[:3]
>>> c[:1:-2]
I really hope you liked this tutorial! π I really appreciate your comments and questions below this post. Please do not hesitate to ask on the discussion forums if you have any questions. Your fellow classmates and Community TAs will be there to help you during this journey.
Estefania.
Great tutorial. Thanks.
ReplyDelete