Iterating over Lists and Tuples: BEHIND THE SCENES!
Hi! Welcome to this section. This time, we will discuss iterables. During your problem sets you will discover the power of iterables and how they can completely change you software development thinking process.
Formally, an iterable is "an object capable of returning its members one at a time . Some data types we have studied are iterables, as you can see in the slide below. Strings and lists and tuples are iterables. And later on you will study Dictionaries (you can iterate over their keys and their corresponding values).
You can find more on iterables in Python's documentation: https://docs.python.org/3.6/glossary.html
But this concept seems so abstract, right? “Capable of returning its members one at a time” What does this mean?
Iterables’ magic comes to life in for loops. Why? Because they return their elements one at a time on every iteration.
This is better illustrated with an example to describe the required syntax:
Iterables in Action!
As you can see, we have a general syntax for using iterables in for loops. But how does this work? What does it do? What will be printed?
Let’s examine this in more detail:
Iterables return their elements one at a time. This means that on every iteration of the for loop, the variable located after the “for” keyword and before the “in” keyword will take the value of an item returned by the iterable. Eventually, when the “for loop” stops executing, this variable will have had as values all the elements in the iterable.
This can be illustrated with an example:
As you can see, the for loop returns the elements in the list from left to right, one at a time on every iteration of the for loop.
The variable before the “in” keyword will take the value of the element returned, BUT ONLY ON ITS CORRESPONDING ITERATION. You can think of this process as if the value of i resets after every iteration and another value is assigned to it.
NOTE: It is important to note that you can name the variable before the “in” keyword anything you’d like. For example, in the slide above we named it “element”. The important fact is to understand that this variable will hold the corresponding value for each iteration and you can use the variable inside the loop.
Here you can see that strings are also iterables, but in this case, their elements are their characters (Like the grid system we studied during String Slicing). On every iteration, the string will return a character from left to right until the variable i has been assigned all the characters in the string.
This process continues until all letters have been evaluated, that is why I use an ellipsis to highlight that the process doesn't end at the last step shown.
Here you can see that tuples are iterables as well.
IMPORTANT: It is important to highlight that the variable before the “in” keyword should be descriptive of a general property of the values it will hold if there is a pattern in the data type elements.
For example, if we have a list of animals, our variable could be names “animal” since it will hold an animal species.
It is also very important to mention that the iterable object can be assigned to a variable before even using it in the “for loop”. In this case, we simply replace the iterable by the variable it’s assigned to.
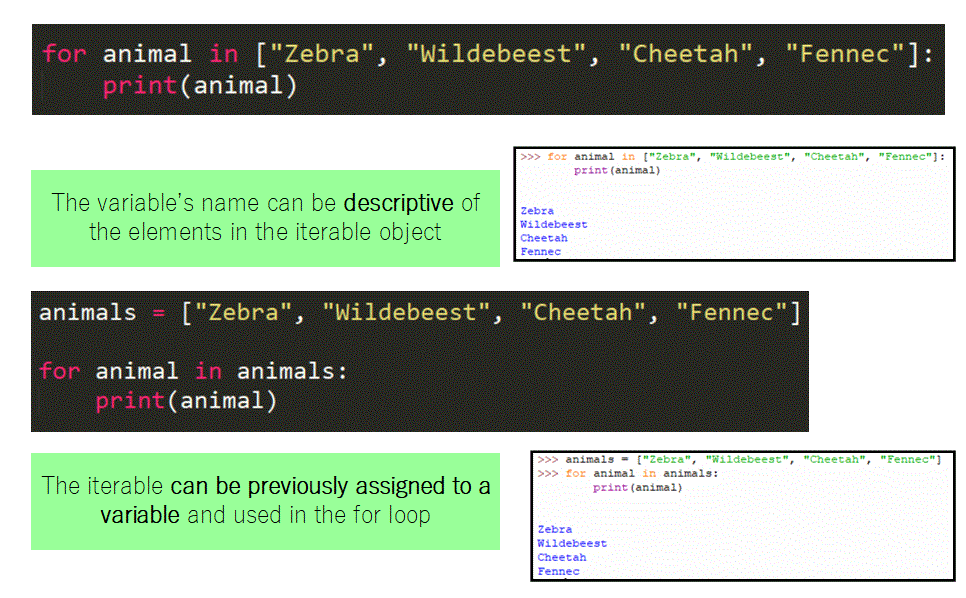
Hope it helps!
If you have any question, please post them in the forums or right below this post, your classmates and Community TAs will always be on the forums to help! :-)
Estefania.
Great info! I recently came across your blog and have been reading along. I thought This is an awesome post.Thanks for this blog,
ReplyDeleteDigital Marketing Training in Chennai
Digital Marketing Course in Chennai